最新要闻
- 灵魂摆渡的作者真的杀了他的妻子吗?灵魂摆渡的经典台词有哪些?
- 董明珠回应直播带货争议:不是为了卖产品 是推广技术
- 当前关注:12万就能上太空!日企将用高空气球开启太空旅游
- 【天天播资讯】昔日国内共享单车巨头!ofo小黄车被曝无法登录:超1600万人押金没退
- “AI飞行员”成功驾驶战斗机 美国顶尖飞行员曾是手下败将
- 播报:暴雪公布《暗黑破坏神4》实机画面:有《暗黑2》那味了
- 六一儿童节的优美句子有哪些?关于六一儿童节的作文汇总
- 移动空调效果如何选择?移动空调哪个牌子好又实惠省电?
- 热文:李楠谈网暴 在线教网友“如何避免成为网暴的施暴者”
- 要闻速递:终于告别“板砖”适配器!联想拯救者Y9000P 2023将支持140W便携快充
- 499元 雷蛇炼狱蝰蛇V3专业版发布:59克超轻量化设计
- 融资客最看好个股一览
- 别乱买!三大要素教你如何选对吸尘器
- 环球看点!一次看个够!《原子之心》双子舞伶所有场景合集
- 世界时讯:微软为Chrome官网注入大幅广告:只为将用户留在Edge
- 天天热资讯!微软收购动视暴雪获新盟友!NVIDIA态度转变签署10年游戏协议
手机
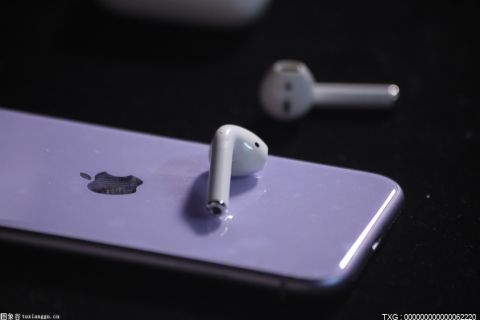
iphone11大小尺寸是多少?苹果iPhone11和iPhone13的区别是什么?

警方通报辅警执法直播中被撞飞:犯罪嫌疑人已投案
- iphone11大小尺寸是多少?苹果iPhone11和iPhone13的区别是什么?
- 警方通报辅警执法直播中被撞飞:犯罪嫌疑人已投案
- 男子被关545天申国赔:获赔18万多 驳回精神抚慰金
- 3天内26名本土感染者,辽宁确诊人数已超安徽
- 广西柳州一男子因纠纷杀害三人后自首
- 洱海坠机4名机组人员被批准为烈士 数千干部群众悼念
家电
世界资讯:单调栈
单调栈
给定一个长度为 N 的整数数列,输出每个数左边第一个比它小的数,如果不存在则输出 −1。
【资料图】
输入格式第一行包含整数 N,表示数列长度。
第二行包含 N 个整数,表示整数数列。
输出格式共一行,包含 N 个整数,其中第 i 个数表示第 i 个数的左边第一个比它小的数,如果不存在则输出 −1。
数据范围\(1≤N≤10^5\)\(1≤数列中元素≤10^9\)输入样例:53 4 2 7 5输出样例:-1 3 -1 2 2
思路
题目需要求每个数左边第一个比它小的数
如果直接暴力的话,时间复杂度是\(o(n^2)\)显然tle了那么我们可以考虑用空间换时间,将之前扫描过的数存储起来
然后我们需要思考的是之前扫描过的什么数对答案是没有贡献的?设\(a_{i-2},a_{i-1},a_i\)为连续的三个数,\(a_i\)为当前枚举到的数显然,如果\(a_{i-2} \ge a_{i-1}\),那么它对答案是没有贡献的(因为如果\(a_{i-1} < a_i\),那么\(a_{i-1}\)就是答案,如果\(a_{i-1} \ge a_i\),那么\(a_{i-2} \ge a_{i-1}\ge a_i\),\(a_{i-2}\)更不可能是答案)
因此,我们只需要维护一个单调递增的栈,每次输出栈顶元素即可
什么是单调栈
栈内元素有单调性,通过维护单调栈实现\(o(n)\)优化
如何维护一个单调栈
单调递增栈:在保持栈内元素单调递增的前提下(如果栈顶元素大于要入栈的元素,将将其弹出),将新元素入栈。
单调递减栈:在保持栈内元素单调递减的前提下(如果栈顶元素小于要入栈的元素,则将其弹出),将新元素入栈。(图片来源:Thr96)
什么时候使用单调栈
给定一个序列,求序列中的每一个数左边或右边第一个比他大或比他小的数在什么地方;
动图演示
以 3 4 2 7 5 为例,过程如下:https://img-blog.csdnimg.cn/20201211221031165.gif#pic_center(动图来源:Hasity)
代码1(stl栈)
点击查看代码
#include#include#include#include#include#include#include#includeusing namespace std;#define X first#define Y secondtypedef pair pii;typedef long long LL;const char nl = "\n";const int N = 1e6+10;const int M = 2e5+10;int n,m;int a[N];stack s;void solve(){cin >> n;for (int i = 1; i <= n; ++i){cin >> a[i];//只用到当前元素while(s.size() && a[i] <= a[s.top()])s.pop(); //弹出栈内所有大于等于当前元素的数,从而维护单调递增序列if(s.size())cout << a[s.top()] << " ";//如果栈不空则输出答案else cout << -1 << " ";//栈空说明答案不存在s.push(i);//当前元素下标入栈}//为什么单调栈储存下标?//因为如果使用下标储存可以通过下标访问数组,如果使用值储存的话不能通过值访问下标(本题用值储存问题也不大,只是为了方便习惯储存下标)}int main(){ios::sync_with_stdio(false);cin.tie(0),cout.tie(0);solve();}
代码2(数组模拟栈)
点击查看代码
#include#include#include#include#include#include#include#includeusing namespace std;#define X first#define Y secondtypedef pair pii;typedef long long LL;const char nl = "\n";const int N = 1e6+10;const int M = 2e5+10;int n,m;int a[N],st[N],tt;void solve(){cin >> n;for (int i = 1; i <= n; ++i){cin >> a[i];while(tt && a[st[tt]] >= a[i])tt --;if(tt)cout << a[st[tt]] << " ";else cout << -1 << " ";st[++ tt] = i;}}int main(){ios::sync_with_stdio(false);cin.tie(0),cout.tie(0);solve();}
一些问题
1. 为什么单调栈储存下标?
因为如果使用下标储存可以通过下标访问数组,如果使用值储存的话不能通过值访问下标(本题用值储存问题也不大,只是为了方便习惯储存下标)
2. 为什么有STL不用,反而要自己用数组来模拟stack?
- 最致命的原因,99%的测评网站,竞赛系统不会对C++代码使用O2优化,导致使用STL的速度慢,容易造成TLE(超时)。
- 学习栈的操作流程和内部原理。
拓展
- 每个数左边第一个比它大的数
点击查看代码
#include#include#include#include#include#include#include#includeusing namespace std;#define X first#define Y secondtypedef pair pii;typedef long long LL;const char nl = "\n";const int N = 1e6+10;const int M = 2e5+10;int n,m;int a[N],st[N],tt;void solve(){cin >> n;for (int i = 1; i <= n; ++i){cin >> a[i];while(tt && a[st[tt]] <= a[i])tt --;if(tt)cout << a[st[tt]] << " ";else cout << -1 << " ";st[++ tt] = i;}}int main(){ios::sync_with_stdio(false);cin.tie(0),cout.tie(0);solve();}
- 每个数右边第一个比它小的数
点击查看代码
#include#include#include#include#include#include#include#includeusing namespace std;#define X first#define Y secondtypedef pair pii;typedef long long LL;const char nl = "\n";const int N = 1e6+10;const int M = 2e5+10;int n,m;int a[N],st[N],tt;vector ans;void solve(){cin >> n;for(int i = 1; i <= n; i ++ )cin >> a[i];for (int i = n; i >= 1; i -- ){while(tt && a[st[tt]] >= a[i])tt --;if(tt)ans.push_back(a[st[tt]]);//cout << a[st[tt]] << " ";else ans.push_back(-1);//cout << -1 << " ";st[++ tt] = i;}for (int i = ans.size() - 1; i >= 0; i -- )cout << ans[i] << " ";}int main(){ios::sync_with_stdio(false);cin.tie(0),cout.tie(0);solve();}
3.每个数右边第一个比它大的数
点击查看代码
#include#include#include#include#include#include#include#includeusing namespace std;#define X first#define Y secondtypedef pair pii;typedef long long LL;const char nl = "\n";const int N = 1e6+10;const int M = 2e5+10;int n,m;int a[N],st[N],tt;vector ans;void solve(){cin >> n;for(int i = 1; i <= n; i ++ )cin >> a[i];for (int i = n; i >= 1; i -- ){while(tt && a[st[tt]] <= a[i])tt --;if(tt)ans.push_back(a[st[tt]]);//cout << a[st[tt]] << " ";else ans.push_back(-1);//cout << -1 << " ";st[++ tt] = i;}for (int i = ans.size() - 1; i >= 0; i -- )cout << ans[i] << " ";}int main(){ios::sync_with_stdio(false);cin.tie(0),cout.tie(0);solve();}
-
-
-
-
世界资讯:单调栈
灵魂摆渡的作者真的杀了他的妻子吗?灵魂摆渡的经典台词有哪些?
董明珠回应直播带货争议:不是为了卖产品 是推广技术
当前关注:12万就能上太空!日企将用高空气球开启太空旅游
【天天播资讯】昔日国内共享单车巨头!ofo小黄车被曝无法登录:超1600万人押金没退
“AI飞行员”成功驾驶战斗机 美国顶尖飞行员曾是手下败将
播报:暴雪公布《暗黑破坏神4》实机画面:有《暗黑2》那味了
六一儿童节的优美句子有哪些?关于六一儿童节的作文汇总
移动空调效果如何选择?移动空调哪个牌子好又实惠省电?
迅雷崩溃是怎么回事?迅雷崩溃怎么解决?
家用数码相机怎么选?家用数码相机什么牌子最好?
三星S8000c什么时候上市的?三星s8000c功能介绍
直播预告 | 企业如何轻松完成数据治理?火山引擎 DataLeap 给你一份实战攻略!
分层测试(五):端到端测试
热文:李楠谈网暴 在线教网友“如何避免成为网暴的施暴者”
要闻速递:终于告别“板砖”适配器!联想拯救者Y9000P 2023将支持140W便携快充
499元 雷蛇炼狱蝰蛇V3专业版发布:59克超轻量化设计
融资客最看好个股一览
【码农教程】手把手教你学会Mockito使用
世界热门:用一个例子学会适配器设计模式
天天视点!量化工具篇
世界播报:2.概率论
视焦点讯!(数据库系统概论|王珊)第六章关系数据理论-第二节:规范化
别乱买!三大要素教你如何选对吸尘器
环球看点!一次看个够!《原子之心》双子舞伶所有场景合集
世界时讯:微软为Chrome官网注入大幅广告:只为将用户留在Edge
天天热资讯!微软收购动视暴雪获新盟友!NVIDIA态度转变签署10年游戏协议
热门:读Java实战(第二版)笔记17_反应式编程
每日播报!若若_关于若若的简介
焦点速读:长沙凌晨1点马路人流量惊人 像在倒时差:网友称想去打卡 当地人回应
全球最资讯丨8GB来了!iPhone 15内存大升级:苹果区别对待 想要花万元买Pro版
环球热头条丨荣耀Magic5 Lite获DXO电池性能第一名 续航可超三天
雷克萨斯、英菲尼迪、凯迪拉克等豪车中国市场暴跌:国人不当冤大头
今日聚焦!面试官:怎么去除 List 中的重复元素?我一行代码搞定,赶紧拿去用!
热文:OPPO打造!唯一配备潜望长焦的天玑旗舰来了
天天短讯!死磕RTX 40!AMD RX 7600/7700/7800齐曝光:据说苏妈定价有诚意
重现《放羊的星星》!林志颖特斯拉车祸后复出晒照 事故原因成谜 是踩错刹车?
Map数据结构详解
环球视讯!在PHP和JavaScript中设置Cookie、会话存储(SessionStorage)和本地存储(LocalStorage)
天天百事通!今日成都到康定怎么坐车_成都到康定
亮机卡也有春天 锐龙7000核显超频到3.1GHz 游戏性能猛增40%
当前要闻:困扰十几亿人!脚趾甲咋会向肉里长?
世界焦点!TCL发布超薄四开门冰箱T9:0cm无缝式嵌入 456L仅3399元
增程、换电、800V高压快充 谁才是未来新能源车最佳补能方式?
环球新动态:发3000元却收回2800元!“慈善主播”被行拘 账号被封
【焦点热闻】秘而不宣的读法
C#的string是一种糟糕的设计吗?
当前视讯!队列——queue的用法(及洛谷B3616)
vue-cli安装依赖 props属性三种方式 混入迷信 插件 elementUI vuex vue Routerd localStorage系列
openfoam文件读取
fusion app自定义事件源码介绍(上)
世界热门:RTX 4070 Laptop逆天能效比!七彩虹将星X15 AT 2023游戏本首发评测
全球快看:1月豪华车销量榜:蔚来碾压全系合资二线豪华品牌
被网友玩坏?微软Bing的ChatGPT被证实变愚蠢了
每日消息!“张伟”骗取数位宝马车主百万购车款 4S店疯狂推卸责任?
AMD锐龙7 7735HS迷你机也有“青春版”:只变了两个USB接口
环球今头条!攒台白色的MATX主机,在B760主板上也能玩好内存超频
全球焦点!RS485 MODBUS转PROFINET网关案例 | 超声波明渠流量计接入到PLC1200 PROFINE
焦点热门:前后端分离项目解决跨域的终极方法
环球今热点:[Java基础]自动装箱与自动拆箱--为什么整型比较必须用equals?
【天天新要闻】Detecting glass in Simulataneous Localisation and Mapping
天天热文:贝叶斯与卡尔曼滤波(2)--连续随机变量的贝叶斯公式
当前热门:用送的那块布擦镜片:小心眼镜被废!
观热点:60岁快递员意外猝死 快递公司回应:深感痛心 善后已达成一致
女子连刷10个差评商家找上门:不好吃你天天来干嘛 谁生活容易啊
【世界报资讯】果粉入手一加Ace 2:开20个应用不杀后台 苹果开3个应用就不行了
80后回忆的“均瑶牛奶”公司进军新能源车:首款纯电SUV云兔来了 莆田生产
信息:Qt调用摄像头一,基础版
k8s多节点二进制部署以及Dashboard UI
记住这12个要点,你也能打造出让HR和技术主管前一亮的前端简历
《原子之心》冰箱诺拉全九国语言配音:怎么没日语?
粉丝发现周深自用手机是iQOO 11 Pro传奇版:5米开外就能看到
电动两轮车总是骑半路就没电?这5种错误充电习惯赶快纠正
世界今头条!男子酷爱嚼槟榔:最终确诊舌癌
【报资讯】免费的ChatGPT意外断网 国内伪装自主的AI露馅了
守护安全|AIRIOT城市天然气综合管理解决方案
0x03_My-OS在实体机上面运行
每日播报!【算法训练营day53】LeetCode1143. 最长公共子序列 LeetCode1035. 不相交的线 LeetCode53. 最大子序和
快讯:两数之和、三数之和、四数之和(双指针)
世界焦点!今日山水一程三生有幸是形容爱情的吗_山水一程三生有幸
环球最资讯丨俞敏洪说想给董宇辉在北京买套房子 这话我听着耳熟
消息!魅族20未发先火!1元超前预订7小时订单破10万
世界热讯:研究称果糖或能导致老年痴呆:专家建议少食用
全球热议:旅日大熊猫香香坐顺丰飞机到家!1个月后与公众见面
环球视讯!成都一公司面试需填芝麻信用分 网友热议
每日信息:100亿级订单怎么调度,来一个大厂的极品方案
[学习笔记]Rocket.Chat业务数据备份
记录--uni-app实现京东canvas拍照识图功能
世界快资讯:Java+Jquer实现趋势图
全球最新:温州特斯拉事故20年驾龄司机仍昏迷:特斯拉回应称难过 重申全力配合调查
环球今热点:Xbox游戏将登陆任天堂主机 网友质疑:NS能带动吗?
世界微资讯!场面爆笑!外国人为开比亚迪开始学中文:难为“小迪”了
即时看!音悦台将回归登热搜!主体公司已成老赖:累计被执行1376万
世界视讯!什么是经营贷什么是消费贷?浅谈二者区别
puppet安装使用踩坑笔记
箭头函数详解
TypeScript 入门自学笔记 — 接口的使用(六)
快资讯丨Python关于异常处理的教程
天天观察:scrollView 嵌套 recyclerview 时 BaseQuickAdapter 九宫格图片拖拽到底部删除
14年老牌网站 音悦台要回归了!官方称很快就要内测